How to Add Links and Form Filling Functionality to Your iOS App with Foxit Mobile PDF SDK
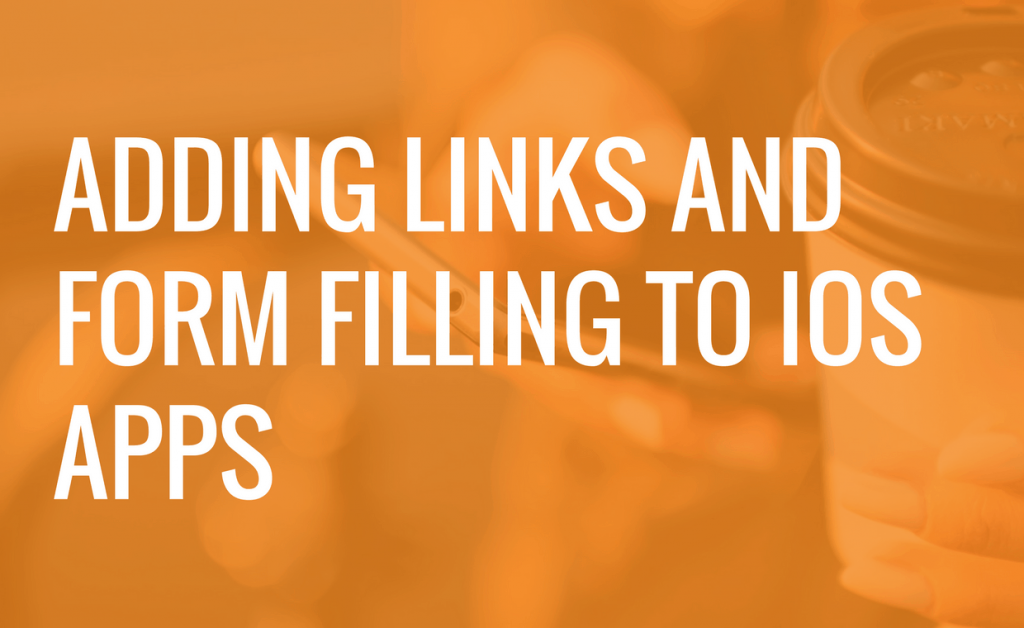
If you’ve purchased (or are considering purchasing) Foxit Mobile PDF SDK, our rapid development kit for mobile platforms, you may be looking forward to the form filling features and ability to add links that comes with it. If so, you’ll be surprised to find out how simple it is to add this functionality.
With just the 4 steps below, you can have full featured forms and links in your iOS app.
Before we jump into the how to guide, it’s important to mention that this guide assumes you’ve already implemented Foxit Mobile PDF SDK and have added the necessary code (literally just a couple of lines) to show PDFs. With that said, let’s get into it:
Contents
1: Add UIExtensions to your project
1a: Import “libFoxitRDKUIExtensions.a”
First, we need to add the extensions library to your project. Do this by right-clicking your project, and selecting Add Files to [your project name here].
After that’s done, add the “-force_load libFoxitRDKUIExtensions.a” to Other Linker Flags in Build Settings.
1b: Add the resource files and the header files
This project only uses “UIExtensionsManager.h”. So you can just add the header file found in the “libs/uiextensions_src/uiextensions” folder of Mobile PDF SDK’s download package to the project
First, copy the uiextensions file from the “libs/uiextensions_src” of Mobile PDF SDK’s download package to your project folder/file. This file contains the header files for the libFoxitRDKUIExtensions.a.
After that, you’ll want to import the resource to the project by right-clicking your project, and selecting Add Files to “[your project name]”. Now you’ve successfully added both the resource and header files to your project.
1c: Rename ViewController.m to ViewController.mm
2: Add the necessary code to your project
Don’t be intimidated by this step as you only need to init the “UIExtensions”. Add this to ViewController.mm.
First, import UIExtensionsManager.h”
#import "UIExtensionsManager.h"
And then Init UIExtensions before show a pdf, the code for this is below:
UIExtensionsManager* extensionsManager;
extensionsManager = [[UIExtensionsManager alloc] initWithPDFViewControl:myTestViewCtrl];
myTestViewCtrl.extensionsManager = extensionsManager;
After that, simply build and run your project. Congratulations, you’ve now implemented form filling functionality and links in your iOS app.
Below is the source code of ViewController.mm with all steps completed:
#import "ViewController.h"
#import <FoxitRDK/FSPDFObjC.h>
#import <FoxitRDK/FSPDFViewControl.h>
#import "UIExtensionsManager.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
NSString* sn = @"";
NSString* unlock = @"";
enum FS_ERRORCODE eRet = [FSLibrary init:sn key:unlock];
if (e_errSuccess != eRet) {
return;
}
//load doc
NSString* docPath= [[NSBundle mainBundle] pathForResource:@"Sample" ofType:@"pdf"];
FSPDFDoc* doc = [FSPDFDoc createFromFilePath:docPath];
if (e_errSuccess!=[doc load:nil]) {
return;
}
//init PDFViewerCtrl
FSPDFViewCtrl* myTestViewCtrl;
myTestViewCtrl = [[FSPDFViewCtrl alloc] initWithFrame:[self.view bounds]];
[myTestViewCtrl setDoc:doc];
[self.view addSubview:myTestViewCtrl];
UIExtensionsManager* extensionsManager;
extensionsManager = [[UIExtensionsManager alloc] initWithPDFViewControl:myTestViewCtrl];
myTestViewCtrl.extensionsManager = extensionsManager;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
We hope this tutorial was useful to you. If you have a project that involves PDF technology in Android or iOS, you should definitely give MobilePDF SDK a try. Try it here for 21 days, for free: http://www.foxit.com/products/sdk/register.php?product=MobilePDFSDK